An Easy Guide to AWS-SDK GO (Golang) on Titan Cloud Storage
Amazon S3 (Simple Storage Service) is a service that Amazon Web Services (AWS) offers to store objects in a scalable, durable, and safe way. Amazon S3 can store and retrieve data anytime, anywhere on the web. It allows users to store and retrieve data from anywhere on the web.
AWS-SDK Go is a software development kit (SDK) for the Go programming language that provides APIs (Application Programming Interfaces) for interacting with various AWS services, including Amazon S3. Using AWS-SDK Go, developers can write Go code to upload, download, and manage objects in Amazon S3.
Titan Cloud Storage is a cloud storage provider that provides an S3-compatible API, meaning developers can use the AWS-SDK Go to interact with Titan Cloud Storage as if it were Amazon S3. Developers can easily move their apps from Amazon S3 to Titan Cloud Storage or use both services since they use the same APIs and tools.
Overall, using the AWS-SDK Go with Titan Cloud Storage provides developers with a flexible, scalable, and cost-effective way to store and manage their data in the cloud.
Prerequisites
Prerequisites required are as follows:
- Go programming language
- AWS SDK for Go package
- A text editor or an IDE
Goals
After finishing this article, you'll be able to:
- Create a new bucket with a specified name.
- Checking if the bucket exists.
- Delete an existing bucket
- Retrieve a list of all buckets in the account.
- Upload a new object to a bucket or replace an existing object with a new version.
- Retrieve an object from a bucket and download it to a local machine.
- Delete an object from a bucket.
- Delete many objects from a bucket.
- Retrieve a list of objects within a bucket.
- Make a copy of an existing object within the same bucket or in a different bucket.
- Check if an object exists in a bucket or not.
- Uploads a file to a bucket.
- Downloads an object from a bucket to a file.
How do I use aws-sdk for Go (Golang) with Titan Cloud Storage
Here are the steps to use Go SDK for Amazon S3 Titan Cloud Storage:
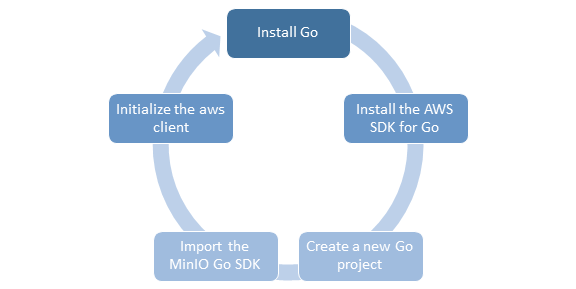
Step 1. Install Go on your system.
• Download and install the Go programming language from the official website at
https://go.dev/doc/install
• To confirm the installation, In Windows, click the Start menu.
• In the menu's search box, type cmd, then press the Enter key.
• Type the following command on cmd.
$ go version

• You're all set to start!
Step 2. Get started with Go
You can start with simple Go code by following steps.
• Create a new Go project. Open a command prompt and make a directory.
mkdir Go-Code
cd Go-Code
We have created a directory on Desktop named “Go-Code”.

For more help, you can also visit https://go.dev/doc/tutorial/getting-started
Step 3. Install the AWS SDK for Go
•
Once you have Go installed, you can install the AWS SDK for Go using the following command:
go get -u github.com/aws/aws-sdk-go

Step 4. Initialize Go project
Run the go mod init command and give it the module's name, which your code will be in. It will create a go.mod file that will track dependencies. The name is the path to the module.
• Initialize it with the following command:go mod init
For Example:go mod init examples/create_connection

Note: A go.mod file that keeps track of the modules that provide those packages defines that module. When your Go example imports packages from other modules, you take care of these dependencies through the module that contains your code. The go.mod file stays with your code, including in your source code repository.
For detail understanding please visit: https://go.dev/ref/mod#go-mod-init
Step 5. Initialize the aws-sdk client and run Go code
• Open text editor, create a file create_connection.go in which to write your code. Paste the following code into your create_connection.go file and save the file.
Go example:
package main
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
// create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
fmt.Println("Connection created successfully.")
// create a s3 client session
titanClient := s3.New(goSession)
// set parameter for bucket name
bucket := aws.String("awssdk-go-bucket")
// create a bucket
_, err = titanClient.CreateBucket(&s3.CreateBucketInput{
Bucket: bucket,
})
// print if there is an error
if err != nil {
fmt.Println(err.Error())
return
}
fmt.Println("Bucket created successfully.")
}
Instance parameters
We have initialized the client ‘titan cloud storage’ in above code by creating a new titan_obj object with the following parameters:
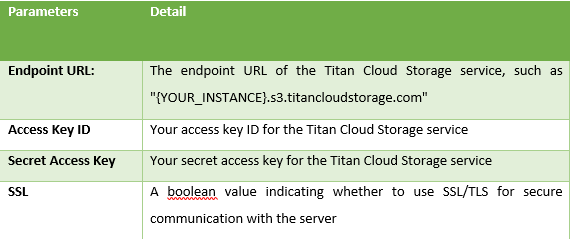
• Run your Go code example.$ go run .
• Output:

These are the basic steps to use the AWS SDK for Go. However, there are many more functions and features available in the SDK that you can use to interact with a wide variety of AWS resources.
Quick Start Examples
With the Aws-sdk Go SDK, developers can quickly and easily build powerful applications that use Titan Cloud Storage's features and take advantage of the Go programming language's ease of use and flexibility. Developers can use the SDK to do many different things with Titan Cloud Storage, like creating and deleting buckets, uploading and downloading objects, and managing bucket policies and metadata.
Bucket operations
Bucket operations are operations that can be performed on buckets in cloud object storage services such as MinIO, Amazon S3, and Google Cloud Storage. Some common bucket operations include:
- Create bucket: Create a new bucket with a specified name.
- Delete bucket: Delete an existing bucket.
- List buckets: Retrieve a list of all buckets in the account.
- Get bucket location: Retrieve the geographic location of a bucket.
- Get bucket object count: Retrieve the number of objects in a bucket.
- Get bucket size: Retrieve the total size of all objects in a bucket.
These operations can be performed using the API provided by the cloud object storage service or using client libraries like MinIO Go. We will see few operations below with code examples and explanation.
1. Initializes a new Titan Cloud Connection Code:
The below code snippets Initializes a new Titan Cloud object storage client.
General Method:
New(endpoint string, opts Options) (Client, error)
The method takes the following parameters:
Code File:
package main
import (
"log"
"github.com/minio/minio-go/v7"
"github.com/minio/minio-go/v7/pkg/credentials"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create a s3 client session
s3Client := s3.New(goSession)
create a configuration
// Code Block 2: set parameter for bucket name and Check if the bucket already exists
bucketName := "no-awssdk-go-bucket"
bucket := aws.String(bucketName)
_, err = s3Client.HeadBucket(&s3.HeadBucketInput{
Bucket: bucket,
})
if err == nil {
fmt.Printf("Bucket '%s' already exists.\n", bucketName)
return
}
}
Run Command:
go run .
2. Check if a bucket exists on Titan Cloud
The below code snippets checks if a bucket exists on the server. It returns True if the bucket exists.General Method:
BucketExists(ctx context.Context, bucketName string) (found bool, err error)
Code File:
package main
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create a s3 client session
s3Client := s3.New(goSession)
create a configuration
// Code Block 2: set parameter for bucket name and Check if the bucket already exists
bucketName := "no-awssdk-go-bucket"
bucket := aws.String(bucketName)
_, err = s3Client.HeadBucket(&s3.HeadBucketInput{
Bucket: bucket,
})
if err == nil {
fmt.Printf("Bucket '%s' already exists.\n", bucketName)
return
}
}
Run Command:
go run .
3. Create a bucket on Titan Cloud
The below code snippets create a bucket on the Titan Cloud server.General Method:
MakeBucket(ctx context.Context, bucketName string, opts MakeBucketOptions)
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
fmt.Println("Connection created successfully.")
// create a s3 client session
titanClient := s3.New(goSession)
// Code Block 2: set parameter for bucket name and create a bucket
bucket := aws.String("awssdk-go-bucket")
_, err = titanClient.CreateBucket(&s3.CreateBucketInput{
Bucket: bucket,
})
// print if there is an error
if err != nil {
fmt.Println(err.Error())
return
}
fmt.Println("Bucket created successfully.")
}
Run Command:
go run .
4. Remove an empty bucket with Titan Cloud Storage
The below code snippets deletes the specified S3 bucket. Before the bucket can be deleted, all of the objects in it, including all versions and delete markers, must be deleted on a Titan Cloud.
General Method:
RemoveBucket(ctx context.Context, bucketName string) error
Code File:
package main
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create an S3 client session
s3Client := s3.New(goSession)
// Code Block 2: set parameter for bucket name and
// check if the bucket already exists then delete the bucket
bucketName := "myawssdk-go-bucket"
, err = s3Client.HeadBucket(&s3.HeadBucketInput{ Bucket: aws.String(bucketName), }) if err != nil { fmt.Printf("Unable to find bucket '%s': %s\n", bucketName, err.Error()) return } // delete the bucket , err = s3Client.DeleteBucket(&s3.DeleteBucketInput{
Bucket: aws.String(bucketName),
})
if err != nil {
fmt.Printf("Unable to delete bucket '%s': %s\n", bucketName, err.Error())
return
}
fmt.Printf("Bucket '%s' deleted successfully.\n", bucketName)
}
Run Command:
go run .
5. List buckets on Titan Cloud Storage
The below code snippets shows all the accessible buckets information on a Titan Cloud.General Method:
ListBuckets(ctx context.Context) ([]BucketInfo, error)
Code File:
package main
import ("fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create a s3 client session
s3Client := s3.New(goSession)
//Code Block 2: List all the buckets in the account.
result, err := s3Client.ListBuckets(&s3.ListBucketsInput{})
if err != nil {
fmt.Println("Error listing buckets:", err)
return
}
if len(result.Buckets) == 0 {
fmt.Println("You don't have any buckets!")
} else {
fmt.Println("Buckets in your account:")
for _, bucket := range result.Buckets {
fmt.Println(*bucket.Name)
}
}
}
Run Command:
go run .
6. Get bucket location, bucket object count and bucket size on Titan Cloud
The below code snippets show two features of bucket information on a Titan Cloud.
- Get Bucket Location
- Get Bucket Count
- Get Bucket Size
1. Get bucket location
"Bucket object count" refers to the total number of objects stored in the bucket. Here's an example of how to use the following code to get the bucket object count the Titan Cloud Storage server using the AWS SDK for Go(Golang).
Code File:
package main
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create an S3 client session
s3Client := s3.New(goSession)
// Code Block 2: list all the buckets in the account and print the count of buckets
result, err := s3Client.ListBuckets(&s3.ListBucketsInput{})
if err != nil {
fmt.Printf("Unable to list buckets: %s\n", err.Error())
return
}
// print the count of buckets
fmt.Printf("Number of buckets: %d\n", len(result.Buckets))
}
Run Command:
go run .
2. Get bucket count
"Bucket size" refers to the total size of all objects stored in the bucket. This can be calculated by adding up the size of each object in the bucket. Here's an example of how to use the following code to get the size of the bucket on the Titan Cloud Storage server using the AWS SDK for Go(Golang).
package main
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create an S3 client session
s3Client := s3.New(goSession)
//Code Block 2: set the name of the bucket whose size you want to check
bucketName := "aws-bucket"
// check if the bucket exists
_, err = s3Client.HeadBucket(&s3.HeadBucketInput{
Bucket: aws.String(bucketName),
})
if err != nil {
fmt.Printf("Unable to find bucket '%s': %s\n", bucketName, err.Error())
return
}
fmt.Printf("Bucket '%s' exists.\n", bucketName)
//Code Block 3: list the objects in the bucket and calculate the total size of the bucket
result, err := s3Client.ListObjectsV2(&s3.ListObjectsV2Input{
Bucket: aws.String(bucketName),
})
if err != nil {
fmt.Printf("Unable to list objects in bucket '%s': %s\n", bucketName, err.Error())
return
}
var totalSize int64
for _, item := range result.Contents {
totalSize += *item.Size
}
fmt.Printf("Size of bucket '%s': %d bytes\n", bucketName, totalSize)
}
Run Command:
go run .
Object operations
Object operations refer to the various actions that can be performed on individual objects stored within a bucket in object storage systems. Some common object operations include:
• Put Object: This operation is used to upload a new object to a bucket or replace an existing object with a new version.
• Get Object: This operation is used to retrieve an object from a bucket and download it to a local machine.
• Delete Object: This operation is used to delete an object from a bucket.
• Delete Multiple Objects: This operation is used to delete many objects from a bucket.
• List Objects: This operation is used to retrieve a list of objects within a bucket.
• Copy Object: This operation is used to make a copy of an existing object within the same bucket or in a different bucket.
• Check Object Existence: This operation is used to check if an object exists in a bucket or not.
1. Copy object to a bucket on Titan Cloud Storage
The code snippets below show how to copy object using AWS SDK for Go(Golang) from a bucket on a Titan Cloud Storage service. Create or replace an object through server-side copying of an existing object. It supports conditional copying, copying a part of an object and server-side encryption of destination and decryption of source.General Method:
CopyObject(ctx context.Context, dst CopyDestOptions, src CopySrcOptions) (UploadInfo, error)
Code File:
package main
import (
"fmt"
"os"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create a s3 client session
s3Client := s3.New(goSession)
//Code Block 2: Set the Source and destination bucket and object to copy then Copy the object
// Source object to copy to
sourceBucket := "my-bucket"
sourceKey := "cat - two.jpg"
// Destination object to copy to
destBucket := "aws-bucket"
destKey := "download/cat - rory.jpg"
// Copy the object
_, err = s3Client.CopyObject(&s3.CopyObjectInput{
Bucket: aws.String(destBucket),
CopySource: aws.String(sourceBucket + "/" + sourceKey),
Key: aws.String(destKey),
})
if err != nil {
fmt.Println("Failed to copy object", err)
os.Exit(1)
}
fmt.Println("Object copied successfully")
}
Run Command:
go run .
2. Check Object Existence in a bucket on Titan Cloud Storage
The code snippets below show how to check object existence using AWS SDK for Go(Golang) from a bucket on a Titan Cloud Storage service.General Method:
StatObject(ctx context.Context, bucketName, objectName string, opts StatObjectOptions) (ObjectInfo, error)
Code File:
package main
import (
"fmt"
"os"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Initialize a new AWS session object.
sess, err := session.NewSession(&aws.Config{
Endpoint: aws.String(endpoint),
Region: aws.String(region),
Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""),
S3ForcePathStyle: aws.Bool(true),
})
if err != nil {
fmt.Println("Failed to create session", err)
os.Exit(1)
}
// Initialize a new S3 client object.
s3Client := s3.New(sess)
//Code Block 2: Set the name of the bucket and check if the object exists in the bucket.
bucketName := "aws-bucket"
objectName := "cat - three.jpg"
_, err = s3Client.HeadObject(&s3.HeadObjectInput{
Bucket: aws.String(bucketName),
Key: aws.String(objectName),
})
if err != nil {
if awsErr, ok := err.(s3.RequestFailure); ok && awsErr.StatusCode() == 404 {
fmt.Printf("Object '%s' does not exist in bucket '%s'.\n", objectName, bucketName)
} else {
fmt.Println("Failed to check object existence", err)
os.Exit(1)
}
} else {
fmt.Printf("Object '%s' exists in bucket '%s'.\n", objectName, bucketName)
}
}
Run Command:
go run .
3. Put Object from a bucket on Titan Cloud
The code snippets below show how to put files from a bucket on a Titan Cloud. The PutObject method is a function provided by the MinIO Go client library that is used to upload an object to a bucket in a Titan Cloud storage service.General Method:
PutObject(ctx context.Context, bucketName, objectName string, reader io.Reader, objectSize int64,opts PutObjectOptions) (info UploadInfo, err error)
Note: Uploads objects that are less than 128MiB in a single PUT operation. For objects that are greater than 128MiB in size, PutObject seamlessly uploads the object as parts of 128MiB or more depending on the actual file size. The max upload size for an object is 5TB.
Code File:
package main
import (
"fmt"
"os"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Initialize a new AWS session object.
session, err := session.NewSession(&aws.Config{
Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""),
Endpoint: aws.String(endpoint),
Region: aws.String(region),
S3ForcePathStyle: aws.Bool(true),
})
if err != nil {
fmt.Println("Error creating session:", err)
return
}
// Create a new S3 client with the session.
s3Client := s3.New(session)
//Code Block 2: Set the name of the bucket and upload the file.
bucketName := "my-bucket"
fileName := "downloadtext.txt"
file, err := os.Open(fileName)
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
// Get the file size and prepare the buffer for the file contents.
fileInfo, err := file.Stat()
if err != nil {
fmt.Println("Error getting file info:", err)
return
}
fileSize := fileInfo.Size()
buffer := make([]byte, fileSize)
// Read the file contents into the buffer.
, err = file.Read(buffer) if err != nil { fmt.Println("Error reading file contents:", err) return } // Upload the file to the bucket. , err = s3Client.PutObject(&s3.PutObjectInput{
Bucket: aws.String(bucketName),
Key: aws.String(fileName),
})
if err != nil {
fmt.Println("Error uploading file:", err)
return
}
fmt.Println("File uploaded successfully.")
}
Run Command:
go run .
4. Get Object from a bucket on Titan Cloud
The code snippets below show how to get object from a bucket on a Titan Cloud.
General Method:
GetObject(ctx context.Context, bucketName, objectName string, opts GetObjectOptions) (*Object, error)
Code File:
package main
import (
"context"
"fmt"
"io"
"log"
"os"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Create a new session with the configuration above
awsConfig := &aws.Config{
Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""),
Endpoint: aws.String(endpoint),
Region: aws.String(region),
S3ForcePathStyle: aws.Bool(true),
}
awsSession, err := session.NewSession(awsConfig)
if err != nil {
log.Fatalln(err)
}
// Initialize a new S3 client object.
s3Client := s3.New(awsSession)
//Code Block 2: Set the name of the bucket and object you want to download.
bucketName := "my-bucket"
objectName := "cat - two.jpg"
// Get information about the object.
object, err := s3Client.GetObjectWithContext(context.Background(), &s3.GetObjectInput{
Bucket: aws.String(bucketName),
Key: aws.String(objectName),
})
if err != nil {
log.Fatalln(err)
}
defer object.Body.Close()
// Create a new file to write the object contents to.
file, err := os.Create(objectName)
if err != nil {
log.Fatalln(err)
}
defer file.Close()
// Write the object contents to the file.
if _, err = io.Copy(file, object.Body); err != nil {
log.Fatalln(err)
}
fmt.Printf("Object '%s' downloaded successfully.\n", objectName)
}
Run Command:
go run .
5. List all the objects in the bucket on Titan Cloud
The code snippets below show how to list the items' details, optionally including bucket versions created on a Titan Cloud.General Method:
ListObjects(ctx context.Context, bucketName string, opts ListObjectsOptions) <-chan ObjectInfo
Code File:
package main
import (
"fmt"
"log"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Create a new AWS session object.
sess, err := session.NewSession(&aws.Config{
Endpoint: aws.String(endpoint),
Region: aws.String(region),
Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""),
S3ForcePathStyle: aws.Bool(true),
})
if err != nil {
log.Fatalln(err)
}
// Create a new S3 client object.
s3Client := s3.New(sess)
//Code Block 2: Set the name of the bucket and list all the objects from the bucket.
bucketName := "my-bucket"
// List all objects in the bucket.
result, err := s3Client.ListObjects(&s3.ListObjectsInput{
Bucket: aws.String(bucketName),
})
if err != nil {
log.Fatalln(err)
}
for _, object := range result.Contents {
fmt.Println(*object.Key, *object.Size, *object.LastModified)
}
}
Run Command:
go run .
6. Remove an object from bucket on Titan Cloud
The below code snippets remove an object from the bucket information on a Titan Cloud.General Method:
RemoveObject(ctx context.Context, bucketName, objectName string, opts minio.RemoveObjectOptions) error
Code File:
package main
import (
"fmt"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
s3Config := aws.Config{
Credentials: credentials.NewStaticCredentials("titanadmin", "TitanDemo123", ""),
Endpoint: aws.String("demo.s3.titancloudstorage.com"),
Region: aws.String("us-east-1"),
S3ForcePathStyle: aws.Bool(true),
}
// create a session with the configuration above
goSession, err := session.NewSessionWithOptions(session.Options{
Config: s3Config,
})
// check if the session was created correctly.
if err != nil {
fmt.Println(err)
}
// create a s3 client session
s3Client := s3.New(goSession)
//Code Block 2: Set the name of the bucket and object and delete the object from the bucket.
deleteObjectInput := &s3.DeleteObjectInput{
Bucket: aws.String("my-bucket"),
Key: aws.String("cat - two.jpg"),
}
// get file
_, err = s3Client.DeleteObject(deleteObjectInput)
fmt.Printf("Object '%s' deleted successfully from bucket '%s'.\n", objectName, bucketName)
}
Run Command:
go run .
7. Remove multiple objects from bucket on Titan Cloud
The code snippets below show how to delete numerous objects from the bucket simultaneously on a Titan Cloud. It removes a list of objects obtained from an input channel.
General Method:
RemoveObjects(ctx context.Context, bucketName string, objectsCh <-chan ObjectInfo, opts RemoveObjectsOptions) <-chan RemoveObjectError
Code File:
package main
import (
"fmt"
"log"
"context"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/credentials"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Create a new AWS session object. sess, err := session.NewSession(&aws.Config{ Endpoint: aws.String(endpoint), Region: aws.String(region), Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""), S3ForcePathStyle: aws.Bool(true), }) if err != nil { log.Fatalln(err) } // Create a new S3 client object. s3Client := s3.New(sess) // Set the name of the bucket and the keys of the objects to remove. bucket := "my-bucket" keys := []string{"articledata.txt", "cat - one.jpg", "downloadtext.txt"} // Create a list of objects to delete. var objects []*s3.ObjectIdentifier for _, key := range keys { objects = append(objects, &s3.ObjectIdentifier{ Key: aws.String(key), }) } // Remove the objects from the bucket. result, err := s3Client.DeleteObjectsWithContext(context.Background(), &s3.DeleteObjectsInput{ Bucket: aws.String(bucket), Delete: &s3.Delete{ Objects: objects, Quiet: aws.Bool(true), }, }) if err != nil { log.Fatalln(err) } fmt.Printf("%d objects deleted successfully from bucket '%s'.\n", len(result.Deleted), bucket)
}
Run Command:
go run .
File Object Operations
In Aws-sdk Go SDK, the file object operations include the following:
• FPutObject - Uploads a file to a bucket.
• FGetObject - Downloads an object from a bucket to a file.
1. Uploads contents from a file to objectName on Titan Cloud Storage
The code snippets below show how to show how to upload contents from a file to objectName using AWS SDK for Go(Golang) from a bucket on a Titan Cloud Storage service.General Method:
FPutObject(ctx context.Context, bucketName, objectName, filePath, opts PutObjectOptions) (info UploadInfo, err error)
Code File:
package main
import (
"context"
"log"
"os"
"github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/aws/session" "github.com/aws/aws-sdk-go/service/s3" "github.com/aws/aws-sdk-go/aws/credentials"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Initialize a new AWS session.
sess, err := session.NewSession(&aws.Config{
Endpoint: aws.String(endpoint),
Region: aws.String(region),
Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""),
S3ForcePathStyle: aws.Bool(true),
})
if err != nil {
log.Fatalln(err)
}
// Create a new S3 client object.
s3Client := s3.New(sess)
// Set the name of the bucket and object you want to upload.
bucketName := "titan-cloud-bucket"
objectName := "articledata.txt"
//Code Block 2: Open the file to upload.
file, err := os.Open(objectName)
if err != nil {
log.Fatalln(err)
}
defer file.Close()
// Get the file information, including its size.
fileStat, err := file.Stat()
if err != nil {
log.Fatalln(err)
}
fileSize := fileStat.Size()
// Upload the file to the bucket.
_, err = s3Client.PutObjectWithContext(context.Background(), &s3.PutObjectInput{
Bucket: aws.String(bucketName),
Key: aws.String(objectName),
Body: file,
})
if err != nil {
log.Fatalln(err)
}
log.Printf("File '%s' uploaded successfully to bucket '%s'. Size: %d bytes.", objectName, bucketName, fileSize)
}
Run Command:
go run .
2. Downloads and saves the object as a file in the local filesystem on Titan Cloud Storage
The code snippets below show how to show downloading and saving the object as a file in the local filesystem using the AWS SDK for Go (Golang) from a bucket on a Titan Cloud Storage service.General Method:
FGetObject(ctx context.Context, bucketName, objectName, filePath string, opts GetObjectOptions) error
Code File:
package main
import (
"context"
"io"
"log"
"os"
"github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/aws/credentials" "github.com/aws/aws-sdk-go/aws/session" "github.com/aws/aws-sdk-go/service/s3"
)
func main() {
//Code Block 1: create a configuration
// Set up credentials and endpoint for Titan Cloud Storage.
endpoint := "demo.s3.titancloudstorage.com"
accessKeyID := "titanadmin"
secretAccessKey := "TitanDemo123"
region := "us-west-1"
// Initialize a new AWS session.
sess, err := session.NewSession(&aws.Config{
Endpoint: aws.String(endpoint),
Region: aws.String(region),
Credentials: credentials.NewStaticCredentials(accessKeyID, secretAccessKey, ""),
S3ForcePathStyle: aws.Bool(true),
})
if err != nil {
log.Fatalln(err)
}
// Create a new S3 client object.
s3Client := s3.New(sess)
//Code Block 2: Set the name of the bucket and object you want to download.
bucketName := "titan-cloud-bucket"
objectName := "articledata.txt"
// Get the current working directory.
currentDir, err := os.Getwd()
if err != nil {
log.Fatalln(err)
}
// Set the path of the file you want to save the object to.
filePath := currentDir + "/download/" + objectName
// Download the object from the bucket.
obj, err := s3Client.GetObjectWithContext(context.Background(), &s3.GetObjectInput{
Bucket: aws.String(bucketName),
Key: aws.String(objectName),
})
if err != nil {
log.Fatalln(err)
}
// Create the file to save the object to.
file, err := os.Create(filePath)
if err != nil {
log.Fatalln(err)
}
defer file.Close()
// Write the object to the file.
_, err = io.Copy(file, obj.Body)
if err != nil {
log.Fatalln(err)
}
log.Printf("Object '%s' downloaded successfully to '%s'.", objectName, filePath)
}
Run Command:
go run .