C# Minio: Utilizing Minio Library for Amazon S3 with Titan Cloud Storage
Amazon S3 is a cloud-based storage service provided by Amazon Web Services (AWS) that allows individuals and businesses to store and retrieve data over the internet. Minio is an open-source, high-performance object storage system that is compatible with Amazon S3 API. This means that developers can use the Minio .NET SDK to build applications that are compatible with Amazon S3 and can store and retrieve data using the Titan Cloud Storage service. Essentially, Minio .NET provides a convenient way for developers to interact with the Titan Cloud Storage service using the same API that Amazon S3 provides, making it easier to build applications that use cloud-based storage.
This quick start guide will show you how to install the Visual studio and run a few basic Go program examples.
Prerequisites
Prerequisites required are as follows:
- Minio .NET SDK package
- Visual Studio IDE
Goals
After finishing this article, you'll be able to:
- Initialize Titan cloud instance.
- Create a new bucket with a specified name.
- Checking if the bucket exists.
- Delete an existing bucket.
- Retrieve a list of all buckets in the account.
- Upload a new object to a bucket or replace an existing object with a new version.
- Retrieve an object from a bucket and download it to a local machine.
- Delete an object from a bucket.
- Delete many objects from a bucket.
- Retrieve a list of objects within a bucket.
- Make a copy of an existing object within the same bucket or in a different bucket.
- Check if an object exists in a bucket or not.
- Uploads a file to a bucket.
- Downloads an object from a bucket to a file.
How do I use Minio for .NET with Titan Cloud Storage
Here are the steps to install and run MinIO SDK for .NET with Visual Studio IDE with Titan Cloud Storage:
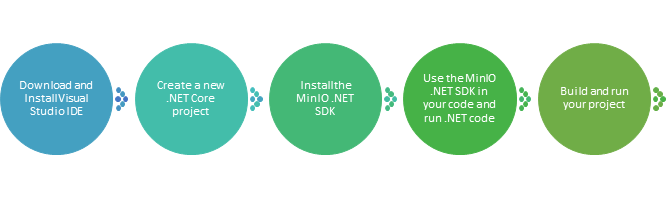
Step 1. Download and Install Visual Studio IDE.
• If you haven't already done so, download and install Visual Studio from the official website based on your operating system.
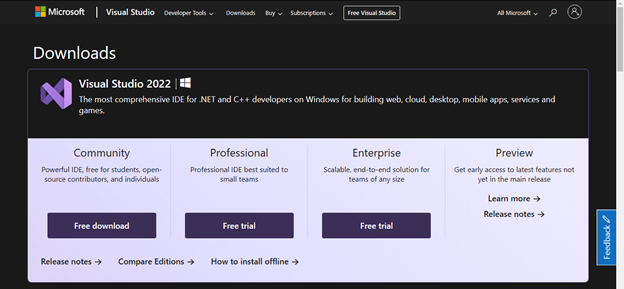
For more details please visit: Download Visual Studio Tools - Install Free for Windows, Mac, Linux (microsoft.com)
Step 2. Create a new .NET Core project
Open Visual Studio and create a new .NET Core console application project by following steps.
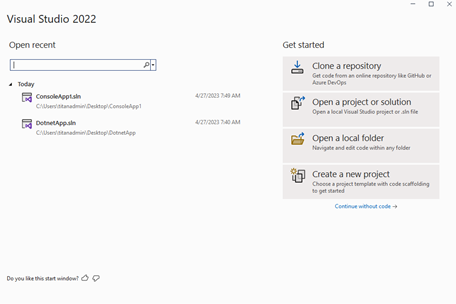
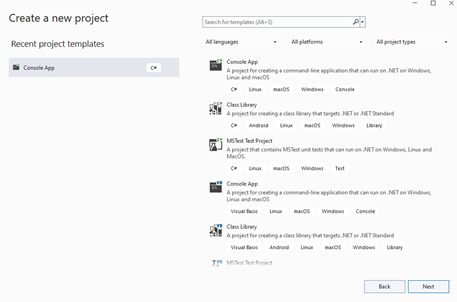
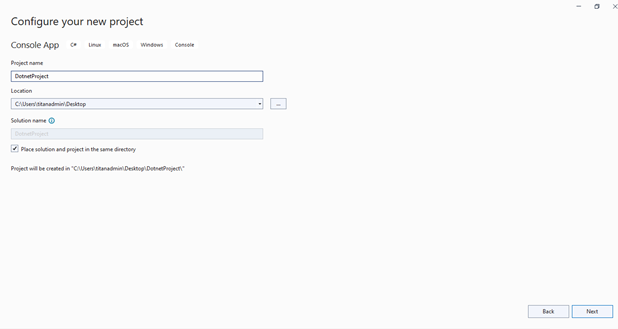
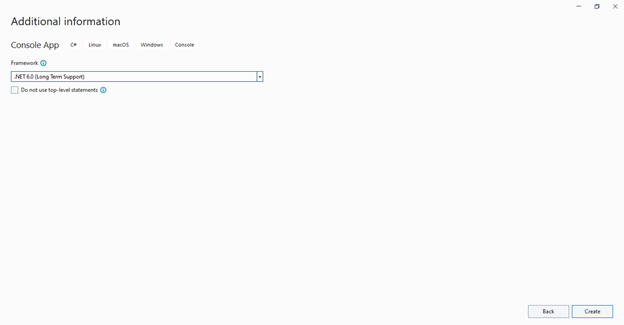
Step 3. Install the MinIO .NET SDK
• Right-click on the project in the Solution Explorer and select "Manage NuGet Packages". Search for "Minio" and install the Minio .NET SDK package.
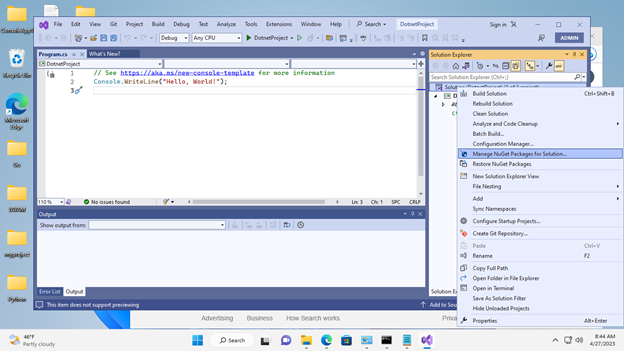
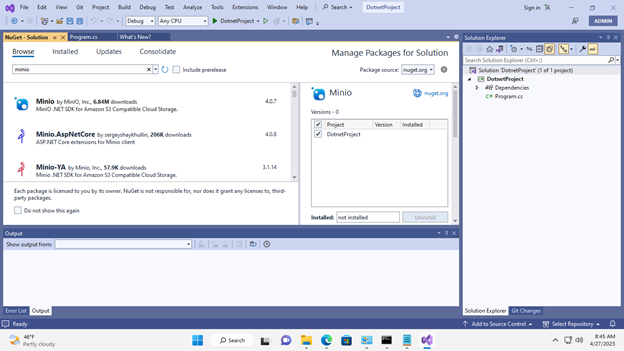
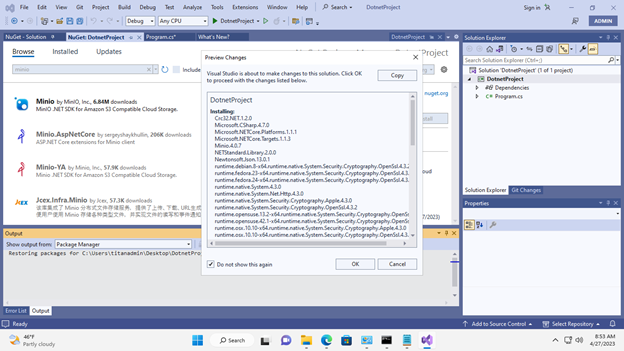
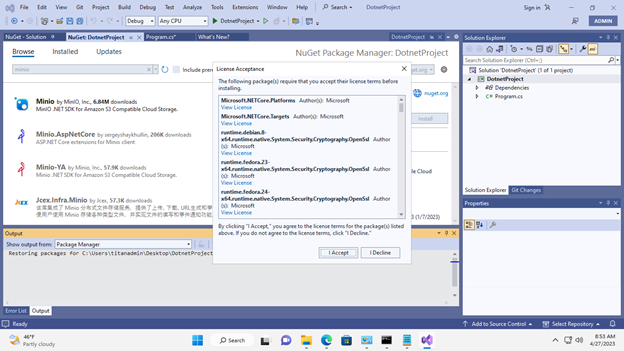
Go and check in Installed tab if the package is installed.
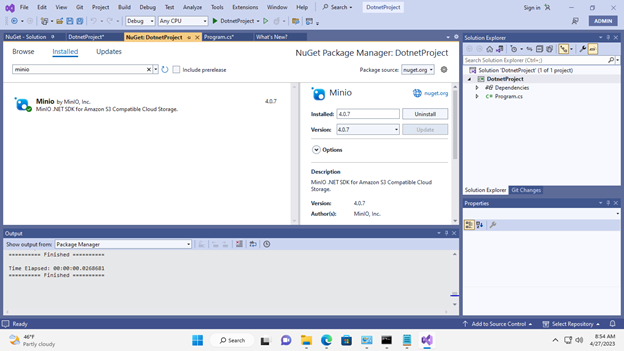
Step 4. Use the MinIO .NET SDK in your code and run .NET code
You can now use the MinIO .NET SDK in your code to interact with a MinIO server. Here's an example of connecting to a MinIO server and listing all buckets:
using Minio;
using System.Net;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var secure = true;
Console.WriteLine("create titan client instance");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Create an async task for listing buckets.
Minio.DataModel.ListAllMyBucketsResult getListBucketsTask = await minio.ListBucketsAsync().ConfigureAwait(false);
// Iterate over the list of buckets.
foreach (var bucket in getListBucketsTask.Buckets)
{
Console.WriteLine(bucket.Name + " " + bucket.CreationDateDateTime);
}
}
}
Step 5. Build and run your project:
Build the application by pressing "Ctrl+Shift+B" or by right-clicking on the project in the Solution Explorer and selecting "Build". Run the application by pressing "F5" or by right-clicking on the project in the Solution Explorer and selecting "Debug > Start New Instance".
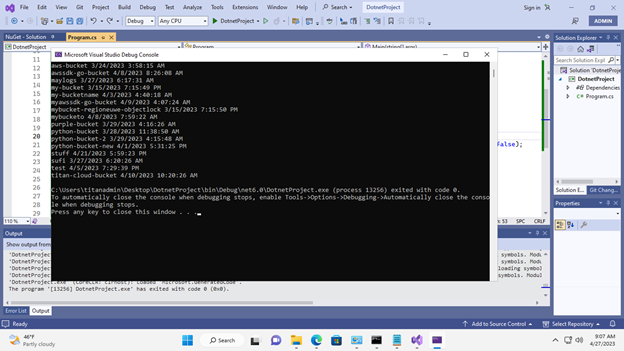
That's it! You now know how to install and run the Minio Client SDK for .NET with Visual Studio.
Quick Start Examples
With the MinIO .NET SDK, developers can quickly and easily build powerful applications that use Titan Cloud Storage's features and take advantage of the .NET programming language's ease of use and flexibility. Developers can use the SDK to do many different things with Titan Cloud Storage, like creating and deleting buckets, uploading and downloading objects, and managing bucket policies and metadata.
Bucket operations
Bucket operations are operations that can be performed on buckets in cloud object storage services such as MinIO, Amazon S3, and Google Cloud Storage. Some common bucket operations include:
• Create bucket: Create a new bucket with a specified name.
• Delete bucket: Delete an existing bucket
• List buckets: Retrieve a list of all buckets in the account.
These operations can be performed using the API provided by the cloud object storage service or using client libraries like Minio Go. We will see few operations below with code examples and explanation.
1. Initializes a new Titan Cloud Connection Code:
The below code snippets Initializes a new Titan Cloud object storage client.
General Method:
public MinioClient(string endpoint, string accessKey = "", string secretKey = "", string region = "", string sessionToken="")
Note: Before running this code, make sure to replace the endpoint
, accessKey
, and secretKey
variables with the appropriate values for your Titan Cloud Storage account.
using Minio;
using Minio.Exceptions;
using System.Net;
using System.Threading.Tasks;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := "<access-key>"
var secretKey := "<secret-key>"
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
Console.ReadLine();
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
2. Check if a bucket exists on Titan Cloud
The below code snippets checks if a bucket exists on the server. It returns True if the bucket exists.General Method:
Task BucketExistsAsync(string bucketName, CancellationToken cancellationToken = default(CancellationToken))
using Minio;
using Minio.Exceptions;
using System.Net;
using System.Threading.Tasks;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := "<access-key>"
var secretKey := "<secret-key>"
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Create a new bucket
var bucketName = "my-dotnet-bucket";
var location = "us-east-1";
var bucketExists = await minio.BucketExistsAsync(bucketName);
try
{
if (!bucketExists)
{
Console.WriteLine($"Bucket '{bucketName}' doesnt exists.");
}
else
{
Console.WriteLine($"Bucket '{bucketName}' already exists.");
}
Console.ReadLine();
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
3. Create a bucket on Titan Cloud
The below code snippets create a bucket on the Titan Cloud server.General Method:
Task MakeBucketAsync(string bucketName, string location = "us-east-1", CancellationToken cancellationToken = default(CancellationToken))
using Minio;
using Minio.Exceptions;
using System.Net;
using System.Threading.Tasks;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := "
var secretKey := "
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Create a new bucket
var bucketName = "my-dotnet-bucket";
var location = "us-east-1";
var bucketExists = await minio.BucketExistsAsync(bucketName);
try
{
if (!bucketExists)
{
await minio.MakeBucketAsync(bucketName, location);
Console.WriteLine($"Bucket '{bucketName}' created successfully.");
}
else
{
Console.WriteLine($"Bucket '{bucketName}' already exists.");
}
Console.ReadLine();
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
4. Remove an empty bucket with Titan Cloud Storage
The below code snippets delete the specified S3 bucket. Before the bucket can be deleted, all of the objects in it, including all versions and delete markers, must be deleted on a Titan Cloud.General Method:
Task RemoveBucketAsync(string bucketName, CancellationToken cancellationToken = default(CancellationToken))
Removes a bucket.
NOTE: - removeBucket does not delete the objects inside the bucket. The objects need to be deleted using the removeObject API.
using Minio;
using Minio.Exceptions;
using System.Net;
using System.Threading.Tasks;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := "
var secretKey := "
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Create a new bucket
var bucketName = "my-dotnet-bucket";
var location = "us-east-1";
var bucketExists = await minio.BucketExistsAsync(bucketName);
try
{
if (!bucketExists)
{
Console.WriteLine($"Bucket '{bucketName}' doesnt exists.");
}
else
{
Console.WriteLine($"Bucket '{bucketName}' exists.");
// Remove bucket my-bucketname. This operation will succeed only if the bucket is empty.
await minio.RemoveBucketAsync(bucketName);
Console.WriteLine($"Bucket '{bucketName}' removed successfully.");
}
Console.ReadLine();
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
5. List buckets on Titan Cloud Storage
The below code snippets show all the accessible buckets information on a Titan Cloud.General Method:
Task ListBucketsAsync(CancellationToken cancellationToken = default(CancellationToken))
using Minio;
using Minio.DataModel;
using Minio.Exceptions;
using System.Net;
using System.Threading.Tasks;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Create a new bucket
var location = "us-east-1";
try
{
var list = await minio.ListBucketsAsync();
// Iterate over the list of buckets.
Console.WriteLine("List of buckets and creation date");
foreach (var bucket in list.Buckets)
{
Console.WriteLine(bucket.Name + " " + bucket.CreationDateDateTime);
}
Console.ReadLine();
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
Object operations
Object operations refer to the various actions that can be performed on individual objects stored within a bucket in object storage systems. Some common object operations include:
• Put Object: This operation is used to upload a new object to a bucket or replace an existing object with a new version.
• Get Object: This operation is used to retrieve an object from a bucket and download it to a local machine.
• Delete Object: This operation is used to delete an object from a bucket.
• Delete Multiple Objects: This operation is used to delete many objects from a bucket.
• List Objects: This operation is used to retrieve a list of objects within a bucket.
• Copy Object: This operation is used to make a copy of an existing object within the same bucket or in a different bucket.
• Check Object Existence: This operation is used to check if an object exists in a bucket or not.
1. Copy object to a bucket on Titan Cloud Storage
The code snippets below show how to copy object using MinIO SDK for .NET from a bucket on a Titan Cloud Storage service. Create or replace an object through server-side copying of an existing object. It supports conditional copying, copying a part of an object and server-side encryption of destination and decryption of source.General Method:
Task CopyObjectAsync(CopyObjectArgs args, CancellationToken cancellationToken = default(CancellationToken))
Code File:
using Minio;
using Minio.Exceptions;
using System;
using System.IO;
using System.Threading.Tasks;
public class Program
{
[Obsolete]
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
var sourceBucket = "my-bucket";
var sourceObject = "cat.jpg";
// name of the destination bucket and object with new name
var destBucket = "aws-bucket";
var destObject = "kitti.jpg";
try
{
// Check if the bucket exists.
bool found = await minio.BucketExistsAsync(sourceBucket);
if (!found)
{
Console.WriteLine($"Bucket '{sourceBucket}' not found.");
return;
}
// copy object to destination with new name
await minio.CopyObjectAsync(sourceBucket, sourceObject, destBucket, destObject).ConfigureAwait(false);
Console.WriteLine($"Object '{sourceObject}' copied to bucket '{destBucket}' with new name '{destObject}'");
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
2. Check Object Existence in a bucket on Titan Cloud Storage
The code snippets below show how to check object existence using MinIO SDK for .NET from a bucket on a Titan Cloud Storage service.General Method:
Task StatObjectAsync(StatObjectArgs args, CancellationToken cancellationToken = default(CancellationToken))
using Minio;
using Minio.Exceptions;
using System;
using System.Threading.Tasks;
public class Program
{
[Obsolete]
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
string bucketName = "my-bucket";
string objectName = "cat.jpg";
bool objectExists = false;
try
{
// Check if object exists in the bucket
var stat = await minio.StatObjectAsync(bucketName, objectName);
objectExists = true;
Console.WriteLine($"Object '{objectName}' exists in the bucket '{bucketName}'.");
}
catch (MinioException e)
{
Console.WriteLine($"Error occurred: {e.Message}");
}
if (!objectExists)
{
Console.WriteLine($"Object '{objectName}' does not exist in the bucket '{bucketName}'.");
}
Console.ReadLine();
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
3. Put Object from a bucket on Titan Cloud
The code snippets below show how to put files from a bucket on a Titan Cloud. The PutObject method is a function provided by the MinIO .NET client library that is used to upload an object to a bucket in a Titan Cloud storage service.General Method:
Task PutObjectAsync(PutObjectArgs args, CancellationToken cancellationToken = default(CancellationToken))
Note: Uploads objects that are less than 128MiB in a single PUT operation. For objects that are greater than 128MiB in size, PutObject seamlessly uploads the object as parts of 128MiB or more depending on the actual file size. The max upload size for an object is 5TB.
Code File:
using Minio;
using Minio.DataModel;
using Minio.Exceptions;
using System;
using System.Threading.Tasks;
public class Program
{
[Obsolete]
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Set the bucket name and object name
var bucketName = "my-bucket";
var objectName = "cat.jpg";
string filePath = Path.Combine(Environment.CurrentDirectory, objectName);
try
{
// Check if the bucket exists.
bool found = await minio.BucketExistsAsync(bucketName);
if (!found)
{
Console.WriteLine($"Bucket '{bucketName}' not found.");
return;
}
// Copy the object from the local file to the specified bucket and object name in Titan Cloud Storage.
await minio.PutObjectAsync(bucketName, objectName, filePath);
Console.WriteLine($"Object '{objectName}' copied to bucket '{bucketName}' in Titan Cloud Storage.");
}
catch (MinioException e)
{
Console.WriteLine($"Error occurred: {e.Message}");
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
4. Get Object from a bucket on Titan Cloud
The code snippets below show how to get object from a bucket on a Titan Cloud. In the MinIO .NET library, the GetObject method is used to retrieve an object from a bucket in the titan cloud storage service.General Method:
Task GetObjectAsync(GetObjectArgs args, ServerSideEncryption sse = null, CancellationToken cancellationToken = default(CancellationToken))
using Minio;
using Minio.Exceptions;
using System;
using System.Threading.Tasks;
public class Program
{
[Obsolete]
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var bucketName = "my-bucket";
var objectName = "cat.jpg";
var localFilePath = "C:\Users\titanadmin\Desktop\DotnetProject\kitti.jpg";
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
try
{
// Check if the bucket exists.
bool found = await minio.BucketExistsAsync(bucketName);
if (!found)
{
Console.WriteLine($"Bucket '{bucketName}' not found.");
return;
}
// Download the object to the current working directory
await minio.GetObjectAsync(bucketName, objectName, localFilePath);
Console.WriteLine($"Object '{objectName}' downloaded to '{localFilePath}'");
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
5. List all the objects in the bucket on Titan Cloud
The code snippets below show how to list the items' details, optionally including bucket versions created on a Titan Cloud.General Method:
ListObjects(ctx context.Context, bucketName string, opts ListObjectsOptions) <-chan ObjectInfo
using Minio;
using Minio.DataModel;
using Minio.Exceptions;
using System;
using System.Collections.Generic;
using System.Reactive.Linq;
using System.Threading.Tasks;
public class Program
{
[Obsolete]
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
Console.WriteLine("Creating Titan instance…\n");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL()
.Build();
string bucketName = "aws-bucket";
bool bucketExists = await minio.BucketExistsAsync(bucketName);
try { if (!bucketExists) { Console.WriteLine($"Bucket '{bucketName}' doesnt exists."); } else { var objectList = new List<string>(); var objects = minio.ListObjectsAsync(bucketName); foreach (var obj in objects) { objectList.Add(obj.Key); } // Print the name of each object foreach (var obj2 in objectList) { Console.WriteLine("Object name: " + obj2.ToString()); } Console.WriteLine("\n "); Console.WriteLine($"Total number of objects: {objectList.Count} in the Bucket '{bucketName}'"); } } catch (MinioException e) { Console.WriteLine("Error occurred: " + e); } }
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
6. Remove an object from bucket on Titan Cloud
The below code snippets remove an object from the bucket information on a Titan Cloud.General Method:
Task RemoveObjectAsync(RemoveObjectArgs args, CancellationToken cancellationToken = default(CancellationToken))
Code File:
using Minio;
using Minio.Exceptions;
using System.Net;
using System.Threading.Tasks;
public class Program
{
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
var secure = true;
Console.WriteLine("create client instance!");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL(secure)
.Build();
// Create a new bucket
var bucketName = "aws-bucket";
var bucketExists = await minio.BucketExistsAsync(bucketName);
try
{
if (!bucketExists)
{
Console.WriteLine($"Bucket '{bucketName}' doesnt exists.");
}
else
{
Console.WriteLine($"Bucket '{bucketName}' exists.");
// Remove the object from the bucket my-bucketname.
RemoveObjectArgs rmArgs = new RemoveObjectArgs()
.WithBucket(bucketName)
.WithObject("kitti.jpg");
await minio.RemoveObjectAsync(rmArgs);
Console.WriteLine($" Object from the Bucket '{bucketName}' removed successfully.");
}
Console.ReadLine();
}
catch (MinioException e)
{
Console.WriteLine("Error occurred: " + e);
}
}
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B
7. Remove multiple objects from bucket on Titan Cloud
7. Remove multiple objects from bucket on Titan Cloud
The code snippets below show how to delete numerous objects from the bucket simultaneously on a Titan Cloud.General Method:
Task> RemoveObjectsAsync(RemoveObjectsArgs args, CancellationToken cancellationToken = default(CancellationToken))
Code File:
using Minio;
using Minio.DataModel;
using Minio.Exceptions;
using System;
using System.Collections.Generic;
using System.Reactive.Linq;
using System.Threading.Tasks;
public class Program
{
[Obsolete]
public static async Task Main(string[] args)
{
var endpoint := "{YOUR_INSTANCE}.s3.titancloudstorage.com"
var accessKey := ""
var secretKey := ""
Console.WriteLine("Creating Titan instance…\n");
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL()
.Build();
string bucketName = "catpix1";
bool bucketExists = await minio.BucketExistsAsync(bucketName);
try { if (!bucketExists) { Console.WriteLine($"Bucket '{bucketName}' doesnt exists."); } else { var objectList = new List<string>(); var objects = minio.ListObjectsAsync(bucketName); foreach (var obj in objects) { objectList.Add(obj.Key); } // Remove specific objects var objectsToRemove = new List<string>() { "example1.mp4", "Pexels Videos 3934.mp4" }; foreach (var obj in objectsToRemove) { await minio.RemoveObjectAsync(bucketName, obj); Console.WriteLine($"Object '{obj}' removed from the bucket '{bucketName}'"); } Console.WriteLine("\n"); // List remaining objects in the bucket objectList.Clear(); objects = minio.ListObjectsAsync(bucketName); foreach (var obj in objects) { objectList.Add(obj.Key); } // Print the name of each object foreach (var obj2 in objectList) { Console.WriteLine("Object name: " + obj2.ToString()); } Console.WriteLine($"\nTotal number of objects: {objectList.Count} in the Bucket '{bucketName}'"); } } catch (MinioException e) { Console.WriteLine("Error occurred: " + e); } }
}
Build Solution:
Right Click on Solution and Click Build Solution or Press Crtl+Shift+B